steve_bank
Diabetic retinopathy and poor eyesight. Typos ...
Lagrange interpretation is easy. Before PCs on everybody's desk I coded it in a programmable calculator to interpolate tables.
In the early days of slow embedded processors interpolation of trig function precalculated tables s could be faster than other means.
You can change he curve and number of points to experiment with it.
en.wikipedia.org
In the early days of slow embedded processors interpolation of trig function precalculated tables s could be faster than other means.
You can change he curve and number of points to experiment with it.
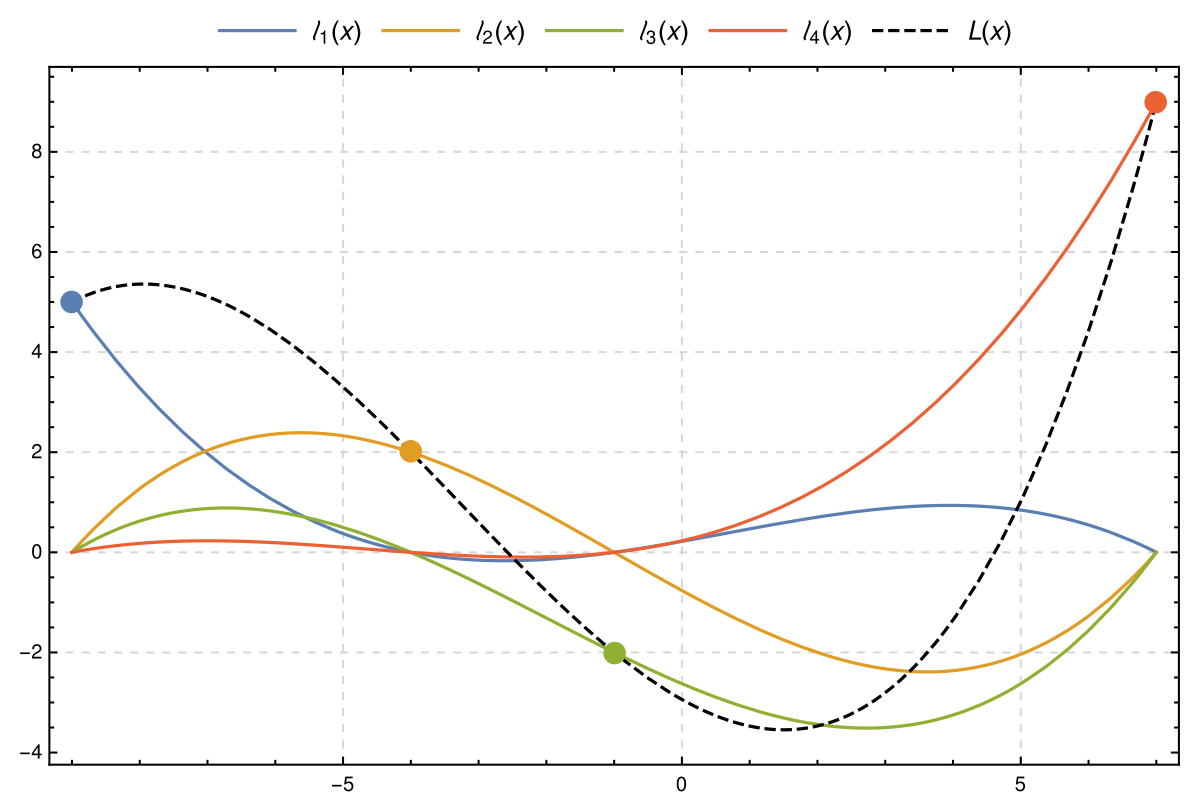
Lagrange polynomial - Wikipedia
Code:
# Python
import math
def lagrange(x,xd,yd):
n = len(xd)
y = 0
for i in range (n):
wp = yd[i]
for j in range(n):
if j != i:
wp = wp * (x - xd[j])/(xd[i] - xd[j])
y += wp
return y
n = 7
xd = n*[0]
yd = n*[0]
lo = 0
hi = math.pi/2 # x range 0 to 90 degrees
dx = (hi-lo)/(n-1)
#ceate data table
for i in range(n):
xd[i] = dx * i
yd[i] = math.sin(xd[i])
xrad = math.pi/7 #x value to be interpolated
xdeg = xrad*180/math.pi
ya = math.sin(xrad) #actual value
y = lagrange(xrad,xd,yd)
print('angle rad-deg ',xrad,' ',xdeg)
print('sim actua-interp ',ya,' ',y)