steve_bank
Diabetic retinopathy and poor eyesight. Typos ...
Calculating e^x
en.wikipedia.org
Scroll down to Representations in the link.
e^x = lim n-> inf (1 + x/n)^n
x = 1 you get e
x = 0 you get 1 ...laws of exponents are satisfied
x = 2
n = 100000000
ex = math.pow(math.e,x) # calculate e^x using Python built in
ep = pow(1. + x/ne,n) # calculate E^x using a method from the link
Result comparing both calculation, a small difference.
7.3890560989306495 7.389056025405986 7.352466369070498e-08
I have no idea what solution Python uses, but it is not using tables.
You really do not know this?
This is not genius level, it is common knowledge in math and engineering.
When I got my first computer in the 80s I went through the books coding in C things like series expansions for math functions. More for understanding than usage. I have always been curious and inquisitive.
Common methods for calculating trig and log functions are on the net. Code in Python and compare to your coding. I would not deprive you of a valuable learning experience.
Python is open sourced, you can find the code that does logs.
The code I posted for the inverse error function is a series.
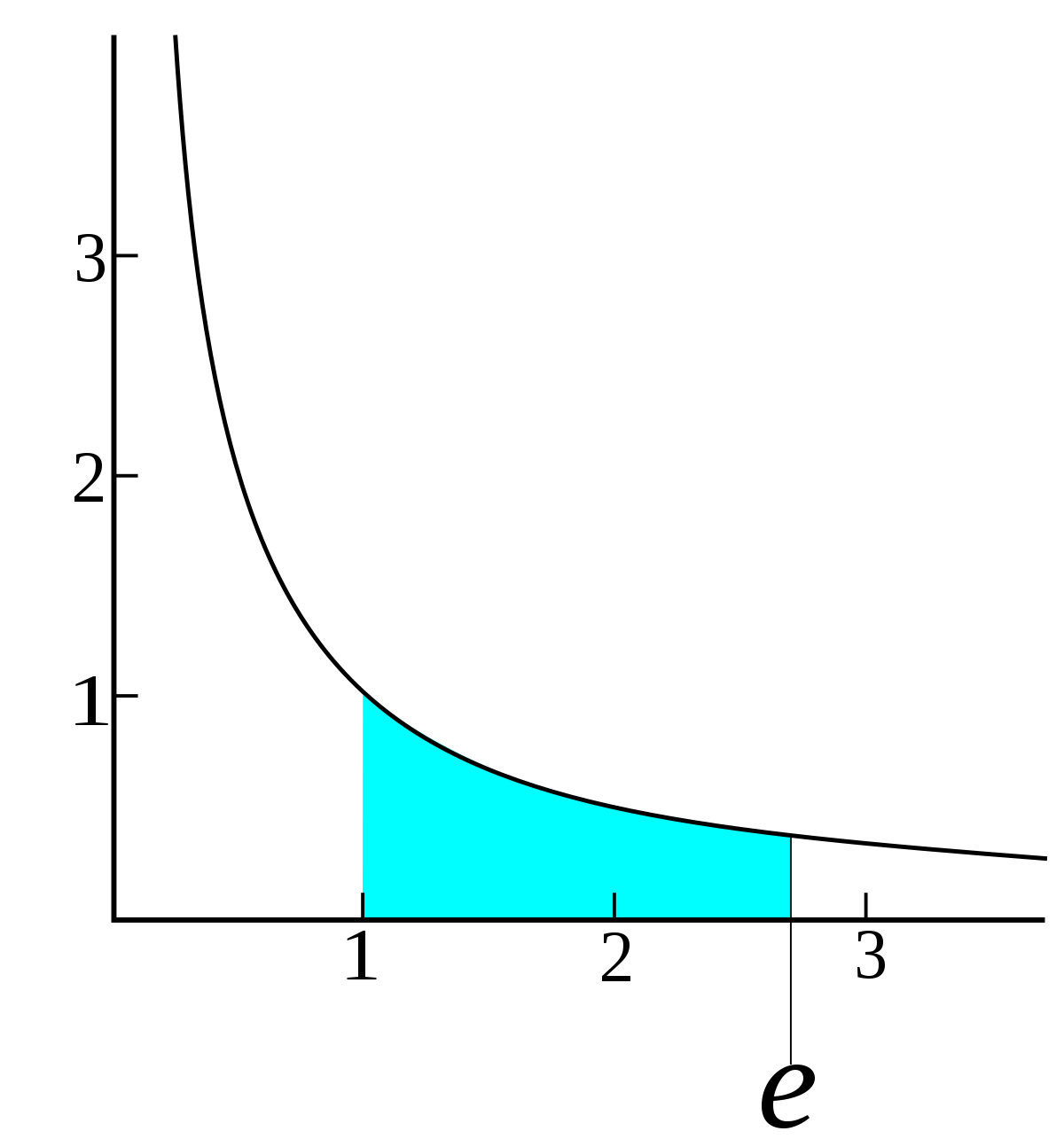
e (mathematical constant) - Wikipedia
Scroll down to Representations in the link.
e^x = lim n-> inf (1 + x/n)^n
x = 1 you get e
x = 0 you get 1 ...laws of exponents are satisfied
x = 2
n = 100000000
ex = math.pow(math.e,x) # calculate e^x using Python built in
ep = pow(1. + x/ne,n) # calculate E^x using a method from the link
Result comparing both calculation, a small difference.
7.3890560989306495 7.389056025405986 7.352466369070498e-08
I have no idea what solution Python uses, but it is not using tables.
You really do not know this?
This is not genius level, it is common knowledge in math and engineering.
When I got my first computer in the 80s I went through the books coding in C things like series expansions for math functions. More for understanding than usage. I have always been curious and inquisitive.
Common methods for calculating trig and log functions are on the net. Code in Python and compare to your coding. I would not deprive you of a valuable learning experience.
Python is open sourced, you can find the code that does logs.
The code I posted for the inverse error function is a series.